Alpine.JS is a popular lightweight JavaScript framework used to create interactive and reactive web interfaces.
In this post, we will discuss four methods to easily add Alpine.JS to Laravel, including directly from the public folder, loading from a CDN URL, loading using Vite, and loading using Laravel Mix.
Let’s get started!
Method 1: Loading Alpine.JS From a CDN URL
This method is useful when you want to load Alpine.JS from a CDN. Simply add the following code to the head section in your blade file:
<script defer src="https://cdn.jsdelivr.net/npm/alpinejs@3.x.x/dist/cdn.min.js"></script>
You can easily test that Alpine.JS works correctly by using this blade code, which makes a dynamic counter based on the example from the Alpine.JS documentation:
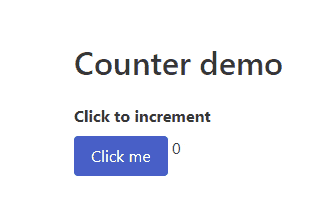
The code to make this example in Laravel blade:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laracoding.com Alpine.JS example</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bulma/0.9.3/css/bulma.min.css">
<script defer src="https://cdn.jsdelivr.net/npm/alpinejs@3.x.x/dist/cdn.min.js"></script>
</head>
<body>
<section class="section">
<div class="container">
<div class="columns">
<div class="column">
<h1 class="title">Counter demo</h1>
<div class="field">
<label class="label">Click to increment</label>
<div class="control">
<div x-data="{ count: 0 }">
<button x-on:click="count++" class="button is-link">Click me</button>
<span x-text="count"></span>
</div>
</div>
</div>
</div>
</div>
</div>
</section>
</body>
</html>
Method 2: Including Alpine.JS Directly From the /public
Folder
This is the simplest method of adding Alpine.JS to Laravel. Follow the steps below:
- Download Alpine.JS from jsdelivr
- Copy the
cdn.min.js
file to thepublic/js
folder of your Laravel application. - Add the following code to the
head
section of your blade file:
<script defer src="{{ asset('js/cdn.min.js') }}"></script>
Method 3: Loading Alpine.JS Using Vite (Laravel 9,10)
Vite is a build tool for modern web applications. Laravel 9 and 10 have in-built support for Vite. Follow the steps below to add Alpine.JS using Vite:
- Install Alpine.JS using NPM by running the following command:
npm install alpinejs
- Create a new file named app.js in the resources/js folder of your Laravel application.
- Add the following code to app.js:
import Alpine from 'alpinejs';
window.Alpine = Alpine;
Alpine.start();
- Add the following code to the
head
section of your blade file.
@vite(['resources/js/app.js'])
Method 4: Loading Alpine.JS Using Laravel Mix (Laravel 5,6,7,8)
Laravel Mix is a wrapper around the popular Webpack module bundler. Follow the steps below to add Alpine.JS using Laravel Mix:
- Install Alpine.JS using NPM by running the following command:
npm install alpinejs
- In your Laravel application, open the webpack.mix.js file.
- Add the following code to the file:
mix.scripts([
'node_modules/alpinejs/dist/cdn.js'
], 'public/js/alpine.js').version();
- Run the following command to compile your assets:
npm run prod
- Add the following code to the
head
section of your blade file:
<script src="{{ mix('js/alpine.js') }}"></script>
Conclusion
In this post, we have discussed four methods to easily add Alpine.JS to Laravel. You can use any of these methods based on your preference and project requirements.
The first two methods are the simplest, but the last two methods are more powerful and provide additional features such as code bundling, minification, and source maps.
Choose the method that best suits your needs and start building awesome interactive web interfaces with Alpine.JS and Laravel.
Now go ahead and add Alpine to your application and create something great. Happy coding!