In Laravel, pagination typically involves querying a database and displaying results in smaller, manageable chunks. However, when working with hardcoded arrays, a different approach is required.
In this tutorial we’ll be using Laravel’s LengthAwarePaginator
class to paginate a hardcoded array.
Interested in paginating Eloquent results instead? Check out my other tutorial: How to Use Pagination in Laravel Blade.
Let’s get started!
Step 1: Create Laravel Project
Begin by creating a new Laravel project named ‘Bookstore’ using the following artisan command:
composer create-project --prefer-dist laravel/laravel Bookstore
Step 2: Create a Controller
Next, create a Controller named BookController
by running:
php artisan make:controller BookController
Step 3: Add Controller Code
Open the generated controller file at app/Http/Controllers/BookController.php
and add the code below.
This code initializes a hardcoded $books
array in the index() method of BookController
. It’s then passed to a paginate()
function to create a LengthAwarePaginator
. Finally, the paginated result is sent to the view returned by the index()
method:
<?php
namespace App\Http\Controllers;
use Illuminate\Contracts\View\View;
use Illuminate\Pagination\LengthAwarePaginator;
class BookController extends Controller
{
/**
* Display a listing of the resource.
*
* @return View The view displaying the list of books.
*/
public function index(): View
{
$books = [
['id' => 1, 'title' => 'Dune'],
['id' => 2, 'title' => 'The Lord of the Rings'],
['id' => 3, 'title' => "Harry Potter and the Philosopher's Stone"],
// ...
];
$paginatedBooks = $this->paginate($books);
return view('books.index', compact('paginatedBooks'));
}
/**
* Paginate an array of items.
*
* @return LengthAwarePaginator The paginated items.
*/
private function paginate(array $items, int $perPage = 5, ?int $page = null, $options = []): LengthAwarePaginator
{
$page = $page ?: (LengthAwarePaginator::resolveCurrentPage() ?: 1);
$items = collect($items);
return new LengthAwarePaginator($items->forPage($page, $perPage), $items->count(), $perPage, $page, $options);
}
}
Step 4: Create a View
Create a file at resources/views/books/index.blade.php
and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Books</title>
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<div class="container mx-auto">
<h1 class="text-3xl font-bold mb-4">Books</h1>
<table class="table-auto w-full border-collapse border border-gray-400">
<thead>
<tr class="bg-gray-200">
<th class="px-4 py-2">Title</th>
<th class="px-4 py-2">Author</th>
</tr>
</thead>
<tbody>
@foreach ($paginatedBooks as $book)
<tr>
<td class="border px-4 py-2">{{ $book['title'] }}</td>
<td class="border px-4 py-2">{{ $book['author'] }}</td>
</tr>
@endforeach
</tbody>
</table>
<div class="mt-8">
{{ $paginatedBooks->withPath(url()->current())->links() }}
</div>
</div>
</body>
</html>
Step 5: Create a Route
Now, open routes/web.php
and add a route for our books page:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\BookController;
// Route to display paginated books
Route::get('/books', [BookController::class, 'index'])->name('books.index');
Step 6: Run the Application
Finally, try out the application by executing the following artisan command:
php artisan serve
Afterwards open your browser and navigate to http://127.0.0.1:8000/books
The result should look like this:
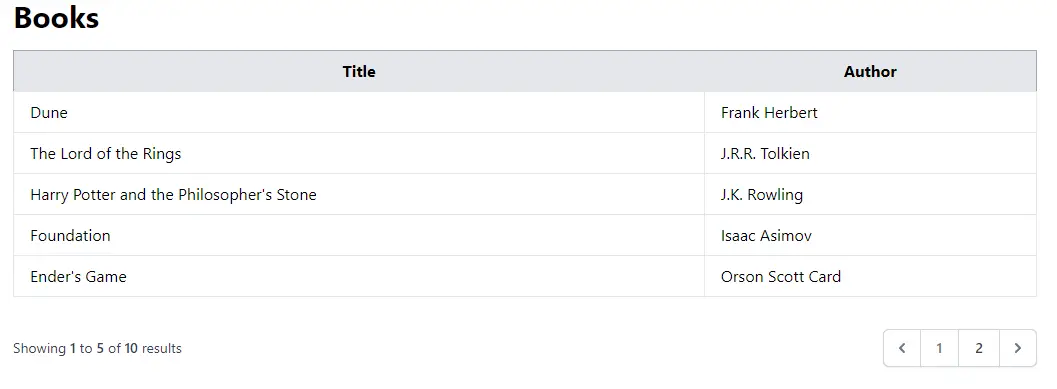
Also try clicking through to the page 2 using the number or the arrowed navigation.
That’s it! You’ve successfully Paginated a hardcoded array using Laravel.
Conclusion
In this tutorial, we explored how to paginate an array of items in a Laravel application. We started by defining a custom pagination method within the controller that uses Laravel’s LengthAwarePaginator
.
We can use this method to paginate any array of items, such as books in our case. By passing the paginated data to the view, we can easily display the items in a paginated format.
Now go ahead and add pagination to present large arrays in a user friendly manner in your own application. Happy Coding!
References
- Manually Creating a Paginator (Laravel Documentation)
- LengthAwarePaginator Class Reference (Laravel API/Illuminate Documentation)
- Tailwind Documentation
Great tutorial,
One question, if we have many records (10k) to pagination, this it the better way to pagination?