Often, we need to populate our database with default data, or we might need a large amount of data for testing our application at scale. Fortunately, Laravel factories allow you to generate any type and quantity of data you may need.
In this guide, we’ll be seeding multiple rows at once into a “products” table by using a model, factory and seeder in conjunction.
Let’s get started!
Step 1: Creating a Laravel Project
Begin by creating a new Laravel project if you haven’t done so already. Open your terminal and run:
composer create-project laravel/laravel product-seeder-app
cd product-seeder-app
Step 2: Creating a Model and Migration
Let’s start by creating our Product model and migration by running:
php artisan make:model Product -m
Step 3: Adding Code to the Migration
Open the newly created migration file and define the up()
and down()
methods as follows:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->decimal('price', 8, 2);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('products');
}
};
Step 4: Running the Migration
To create the table, as defined in our migration, run the following artisan command:
php artisan migrate
Step 5: Creating a Factory
Next, create a factory for the Product model to generate sample data:
php artisan make:factory ProductFactory --model=Product
This command generates the ProductFactory.php
file within the database/factories
directory.
Step 6: Adding Code to the Factory
Within the ProductFactory.php
file, define the attributes and their respective fake random data.
use App\Models\Product;
use Illuminate\Database\Eloquent\Factories\Factory;
class ProductFactory extends Factory
{
protected $model = Product::class;
public function definition()
{
return [
'name' => $this->faker->sentence(2),
'description' => $this->faker->sentence(4),
'price' => $this->faker->randomFloat(2, 10, 1000),
];
}
}
Step 7: Seeding Multiple Rows
Now, let’s create a seeder to seed multiple rows using the factory. Generate a seeder for the Product model:
php artisan make:seeder ProductsTableSeeder
In the ProductsTableSeeder.php
file, use the factory to seed multiple rows:
<?php
namespace Database\Seeders;
use App\Models\Product;
use Illuminate\Database\Seeder;
class ProductsTableSeeder extends Seeder
{
/**
* Run the database seeds.
*/
public function run(): void
{
Product::factory()->count(10)->create();
}
}
Step 8: Running the Seeder
Now let’s run our seeder by using the following artisan command:
php artisan db:seed --class=ProductsTableSeeder
Step 9: Check data in the database
Now we can verify we have 10 products generated by looking in our mysql database:
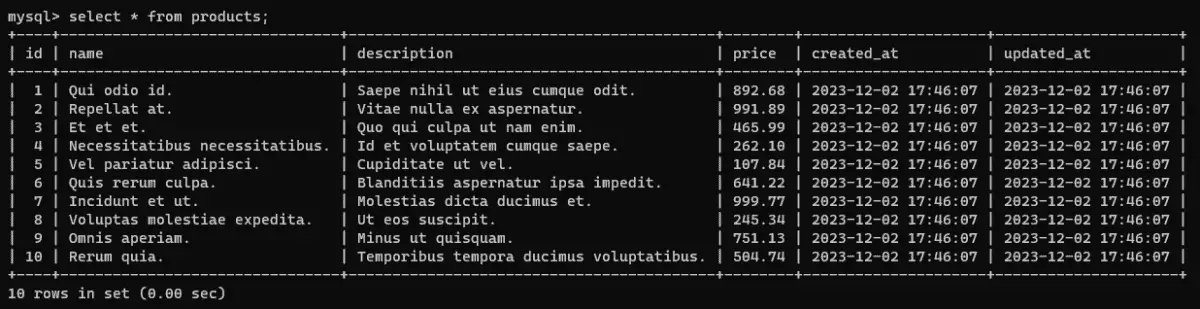
In this case, I used the command line mysql client to check the table’s content. You may also use other free tools like Heidi SQL (Windows), Sequel ACE (MAC).
That’s it! We’ve successfully generated 10 records of data. We can easily generate more by increasing the number in our ProductsTableSeeder
.
Product::factory()->count(100000)->create(); // Generate a hundred thousand records
Conclusion
By following the steps in this tutorial, you’ve successfully set up a model, migration, factory, and seeder to seed multiple rows based on the Product Model using Laravel factories. This approach allows you to fill your database with realistic data, saving time and effort during development.
Happy coding!
Note: Factories also support seeding models with Relationships. Read our Guide Seeding a Model With Relationship Using Laravel Factories to learn more.
References:
- Database Seeding (Laravel Documentation)
- Eloquent Factories (Laravel Documentation)