Writing your own JavaScript is often needed to add reactivity to your Laravel application. By combining the strengths of Laravel and Vite, you can effortlessly include and manage your custom JavaScript, ensuring a well-optimized, smooth, and dynamic user experience.
In this tutorial, I’ll walk you through the steps involved. Let’s dive right in!
Step 1: Create a New Laravel Project
If you haven’t already, let’s start by creating a new Laravel project. Open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel my-laravel-app
cd my-laravel-app
Step 2: Create Your Custom JavaScript File
Inside your Vite project directory, navigate to the src
folder and create a new JavaScript file for your custom code. For example, let’s call it my-custom.js
. You can use any code editor to create this file.
document.addEventListener('DOMContentLoaded', function() {
const customElement = document.createElement('div');
customElement.innerText = 'Custom JavaScript is running!';
customElement.style.backgroundColor = 'lightgreen';
customElement.style.padding = '10px';
customElement.style.textAlign = 'center';
document.body.appendChild(customElement);
});
Step 3: Import Your Custom JavaScript in app.js
Open the resources/js/app.js
file in your Laravel project, and import your custom JavaScript file at the end of the file:
// Import default Laravel bootstrapper, adds axios
import './bootstrap';
// Import our own JavaScript located in resources/js/my-custom.js
import './my-custom';
Step 4: Edit Blade Template to Add @vite
Directive
To make sure your custom JavaScript is included in your Blade templates, add the @vite
directive within the HTML <head>
section. For example, in resources/views/welcome.blade.php
:
<!DOCTYPE html>
<html>
<head>
<!-- ... -->
@vite(['resources/js/app.js'])
</head>
<body>
<!-- ... -->
</body>
</html>
This directive tells Vite to include your custom JavaScript in the rendered HTML.
Step 5: Compile Your Assets
Next, compile your assets using Vite. To do this, run the following command:
For development purposes, use:
npm run dev
For production, use:
npm run build
In both cases, the JavaScript is compiled into the folder public/build
. However, there are differences:
- The
run dev
version stays running and recompiles when it detects changed files. Note thatrun dev
will not minify your code. - The
run build
version compiles runs just once and closes. Note thatrun build
minifies the code ensuring it is optimized for production use.
Step 6: Test Your Custom JavaScript
To test your custom JavaScript, start the Laravel development server:
php artisan serve
Open your browser and navigate to your Laravel application. Your custom JavaScript should now be loaded and functioning, which you can see by the green bar we’ve drawn using my-custom.js:
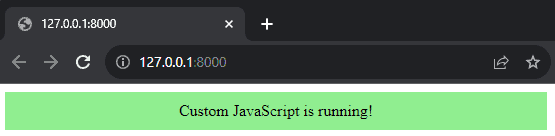
That’s it! You’ve successfully included a custom JavaScript file and compiled it into the application’s public app.js
.
Advanced Tips Using Custom JS With Vite
Tip 1: Import Custom JS Functions Into the Global window
Object
We can export a library of our custom functions and then import and assign them to the global window object. This way we can use them anywhere in the application. To do this follow the following steps:
- Create
resources/js/utilities.js
and add:
export function greet(name) {
return `Hello, ${name}!`;
}
export function add(a, b) {
return a + b;
}
- Edit
resources/js/app.js
and add the import:
// Import default Laravel bootstrapper, which adds axios
import './bootstrap';
// Import custom JavaScript functions from resources/js/utilities.js
import { greet, add } from './utilities';
window.greet = greet;
window.add = add;
- Edit your code in
resources/views/welcome.blade.php
as follows:
<html>
<head>
<!-- .. other head code -->
@vite(['resources/js/app.js'])
</head>
<body>
<!-- .. other body code -->
<script type="module">
// Call the imported functions
const greeting = greet('John');
console.log(greeting); // Output: 'Hello, John!'
const sum = add(3, 5);
console.log('3 + 5 = ' + sum); // Output: "3 + 5 = 8"
</script>
</body>
</html>
Note that we added type=”module” to the script tag. If we don’t add it the browser will show an error in the console “Uncaught ReferenceError: greet is not defined”.
Tip 2: Import Custom JS Functions Into a Custom Object
To improve the structure of your code you can bundle multiple custom functions into a custom object. Then you can access the functions contained in the object with a convenient dot syntax.
In other words, we’ll be able to write for example: MyUtil.greet('John')
or MyUtil.add(3, 5);
To achieve this with Vite follow these steps:
- Create
resources/js/utilities.js
and add:
export function greet(name) {
return `Hello, ${name}!`;
}
export function add(a, b) {
return a + b;
}
- Edit
resources/js/app.js
and add the import:
// Import default Laravel bootstrapper, which adds axios
import './bootstrap';
// Import functions
import * as MyUtil from './utilities'
window.MyUtil = MyUtil;
- Edit your code in
resources/views/welcome.blade.php
as follows:
<html>
<head>
<!-- ... -->
@vite(['resources/js/app.js'])
</head>
<body>
<!-- ... -->
<script type="module">
const greeting = MyUtil.greet('John');
const sum = MyUtil.add(3, 5);
console.log(greeting); // Output: "Hello, John!"
console.log('3 + 5 = ' + sum); // Output: "3 + 5 = 8"
</script>
</body>
</html>
Note that we added type=”module” to the script tag. If we don’t add it the browser will show an error in the console “Uncaught ReferenceError: MyUtil is not defined”.
Conclusion
By following these steps, you’ve successfully added custom JavaScript to your Laravel application using Vite. This allows you to enhance the functionality and interactivity of your web application efficiently.
Furthermore, the techniques demonstrated in the advanced tips enable you to better reuse your custom functions throughout your application and wrapping the functions in custom objects will contribute to improved organization of your code.
References
- Loading Scripts and Styles With Vite (Laravel Documentation)
This entry is part 5 of 5 in the series Installing Laravel Assets Using Vite
- How to Install Font Awesome in Laravel With Vite
- How to Install jQuery in Laravel With Vite
- How to Install Bootstrap in Laravel With Vite
- How to Install TinyMCE in Laravel With Vite
- How to Add Custom JavaScript to Laravel With Vite
Thank you 🙏
You’re most welcome. I’m glad you’ve found the post helpful 🙂
Thanks a lot, Johan.
You’re welcome and happy coding! 🙂
finally i founded, thank you sir
You’re welcome, I’m glad to help! 🙂