Bootstrap is a popular front-end framework that helps build applications with a decent look and feel while supporting various screen sizes found on desktops, phones, and other devices. It can be installed in your Laravel application in numerous ways but using Vite for this has certain advantages.
Vite has been an integral part of Laravel since version 8, serving as the default build tool. By leveraging Vite to compile your CSS and JS, along with dependencies like Bootstrap, you can easily optimize and minify your assets.
In this step-by-step guide, I’ll walk you through the process of adding Bootstrap to your Laravel project using Vite, a powerful build tool that ships with Laravel. Let’s get started!
Step 1: Create a New Laravel Project
Start by creating a new Laravel project. Open your terminal and run the following commands:
composer create-project laravel/laravel laravel-bootstrap-vite
cd laravel-bootstrap-vite
Step 2: Use npm to install bootstrap + popperjs + sass
To use Bootstrap 5 in your Laravel project, you should install Bootstrap, Popper JS, and SASS by running the following command:
npm install bootstrap @popperjs/core sass
We need popperjs to support popover features of Bootstrap, like dropdowns and hamburger menus. The support of SASS is needed so that Vite can compile Bootstraps’ SCSS files to regular CSS and provide them in the public folder, as required by the browsers visiting your site.
Step 3: Configure Vite for Laravel
Now, it’s time to configure Vite for your Laravel project. Open the vite.config.js
file and use the following code:
import { defineConfig } from 'vite';
import laravel from 'laravel-vite-plugin';
export default defineConfig({
plugins: [
laravel({
input: [
'resources/sass/app.scss',
'resources/js/app.js',
],
refresh: true,
}),
]
});
This configuration ensures that Vite processes your project’s assets correctly when making the asset builds with either npm or yarn.
Step 4: Update the File app.scss
Edit the resources/sass/app.scss
file to include Bootstrap and any custom styles you want to add. For example:
// Fonts
@import url('https://fonts.bunny.net/css?family=Nunito');
// Bootstrap
@import 'bootstrap/scss/bootstrap';
Step 5: Update the File app.js
Edit the resources/sass/app.js
file and add an import statement for adding Bootstraps JavaScript. It should look like somewhat like the following:
// Default Laravel bootstrapper, installs axios
import './bootstrap';
// Added: Actual Bootstrap JavaScript dependency
import 'bootstrap';
// Added: Popper.js dependency for popover support in Bootstrap
import '@popperjs/core';
Step 6: Modify the Blade Template
Open the welcome.blade.php
file and add the @vite
directive to the head section as shown below.
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<!-- .. Other head code -->
<!-- Scripts -->
@vite(['resources/sass/app.scss', 'resources/js/app.js'])
</head>
<body>
<main>
<!-- .. Main HTML -->
</main>
</body>
</html>
Now with this code in place, you can use any components and features supported in Bootstrap 5.
As an example, I’ve included an example layout based on the official Bootstrap 5 documentation’s Jumbotron for you to use:
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- CSRF Token -->
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<!-- Scripts -->
@vite(['resources/sass/app.scss', 'resources/js/app.js'])
</head>
<body>
<main>
<div class="container py-4">
<header class="pb-3 mb-4 border-bottom">
<a href="/" class="d-flex align-items-center text-body-emphasis text-decoration-none">
<svg xmlns="http://www.w3.org/2000/svg" width="40" height="32" class="me-2" viewBox="0 0 118 94" role="img"><title>Bootstrap</title><path fill-rule="evenodd" clip-rule="evenodd" d="M24.509 0c-6.733 0-11.715 5.893-11.492 12.284.214 6.14-.064 14.092-2.066 20.577C8.943 39.365 5.547 43.485 0 44.014v5.972c5.547.529 8.943 4.649 10.951 11.153 2.002 6.485 2.28 14.437 2.066 20.577C12.794 88.106 17.776 94 24.51 94H93.5c6.733 0 11.714-5.893 11.491-12.284-.214-6.14.064-14.092 2.066-20.577 2.009-6.504 5.396-10.624 10.943-11.153v-5.972c-5.547-.529-8.934-4.649-10.943-11.153-2.002-6.484-2.28-14.437-2.066-20.577C105.214 5.894 100.233 0 93.5 0H24.508zM80 57.863C80 66.663 73.436 72 62.543 72H44a2 2 0 01-2-2V24a2 2 0 012-2h18.437c9.083 0 15.044 4.92 15.044 12.474 0 5.302-4.01 10.049-9.119 10.88v.277C75.317 46.394 80 51.21 80 57.863zM60.521 28.34H49.948v14.934h8.905c6.884 0 10.68-2.772 10.68-7.727 0-4.643-3.264-7.207-9.012-7.207zM49.948 49.2v16.458H60.91c7.167 0 10.964-2.876 10.964-8.281 0-5.406-3.903-8.178-11.425-8.178H49.948z" fill="currentColor"></path></svg>
<span class="fs-4">Laracoding.com Tutorial</span>
</a>
</header>
<div class="p-5 mb-4 bg-body-tertiary rounded-3">
<div class="container-fluid py-5">
<h1 class="display-5 fw-bold">Laravel/Vite Bootstrap 5 Example</h1>
<p class="col-md-8 fs-4">This simple jumbotron example is based on one of the official Bootstrap 5 examples.</p>
<a class="btn btn-primary btn-lg" href="https://getbootstrap.com/docs/5.3/examples/jumbotron/">
View on getbootstrap.com
</a>
</div>
</div>
<div class="row align-items-md-stretch">
<div class="col-md-6">
<div class="h-100 p-5 text-bg-dark rounded-3">
<h2>Dark background</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat..</p>
<button class="btn btn-outline-light" type="button">Example button</button>
</div>
</div>
<div class="col-md-6">
<div class="h-100 p-5 bg-body-tertiary border rounded-3">
<h2>Some borders</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat..</p>
<button class="btn btn-outline-secondary" type="button">Example button</button>
</div>
</div>
</div>
<footer class="pt-3 mt-4 text-body-secondary border-top">
© 2023
</footer>
</div>
</main>
</body>
</html>
Step 7: Run Your Laravel Application
Now that you’ve configured everything, run your Laravel application with the following command:
php artisan serve
Open your web browser and navigate to the URL provided by Laravel. You’ll see your Laravel project with Bootstrap 5 styles applied. I should look like the following:
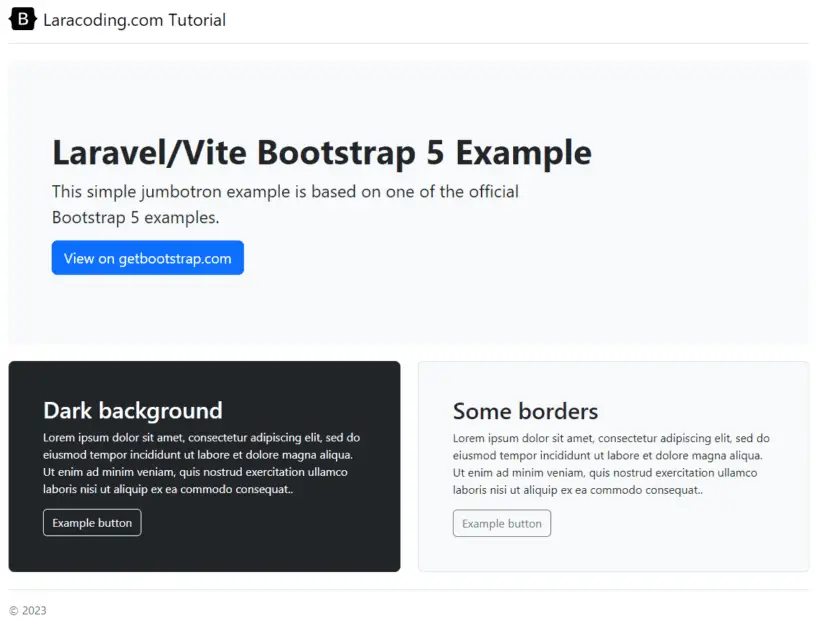
Frequently Asked Questions
How can I Install and Use Bootstrap Icons in Laravel with Vite?
First instruct npm to download the current bootstrap-icons release by running:
npm install bootstrap-icons
Then add an import for bootstrap-icons in your resources/sass/app.scss
. It should look like the following:
// Fonts
@import url('https://fonts.bunny.net/css?family=Nunito');
// Bootstrap
@import 'bootstrap/scss/bootstrap';
// Added: Bootstrap icons
@import 'bootstrap-icons/font/bootstrap-icons.css';
Run:
npm run dev
Now you can use bootstrap icons in your code like:
<i class="bi bi-bag-heart-fill"></i>
<i class="bi bi-app"></i>
<i class="bi bi-arrow-right-square-fill"></i>
<i class="bi bi-bag-check-fill"></i>
<i class="bi bi-calendar-plus-fill"></i>
<i class="bi bi-airplane"></i>
<i class="bi bi-bar-chart-fill"></i>
<i class="bi bi-bell-fill"></i>
This looks like:

For a list of all supported icons and their codes go to: icons.getbootstrap.com
You can also use Font Awesome or you could even use both at the same time. To learn how to add Font Awesome (optional), read our guide: how to install Font Awesome in Laravel with Vite
How Can I Update the Bootstrap Version Using Vite?
One advantage of using Vite for asset management is its ease of updating dependencies. When Bootstrap releases a new version, you can update and recompile your assets by running these simple commands:
npm update bootstrap
npm run build
That’s it! You’re now using the most recent Bootstrap version.
How can I Install a Specific Version of Bootstrap Using Vite?
In case you’d like to use a lower version than the current Bootstrap release you can install a specific version by running:
npm install bootstrap@4.6
npm run build
Conclusion
You’ve successfully integrated Bootstrap 5 into your Laravel project using Vite. With Bootstrap’s powerful features and Vite’s asset compilation, you can create applications that load fast and look professional.
Finally, within the FAQ section, we’ve addressed some key topics. Firstly, I’ve covered how you can add Bootstrap icons. Lastly, we’ve provided insights on how to update or install a specific version of Bootstrap.
Happy coding!
References
This entry is part 3 of 5 in the series Installing Laravel Assets Using Vite
- How to Install Font Awesome in Laravel With Vite
- How to Install jQuery in Laravel With Vite
- How to Install Bootstrap in Laravel With Vite
- How to Install TinyMCE in Laravel With Vite
- How to Add Custom JavaScript to Laravel With Vite
Why are you importing the font in your scss file and then linking the font in the blade template? Isn’t that redundant?
Indeed that’s redundant. I’ve corrected the issue by removing the font inclusion from the blade code while keeping it in he scss.
Nicely spotted and thanks for pointing out this mistake!